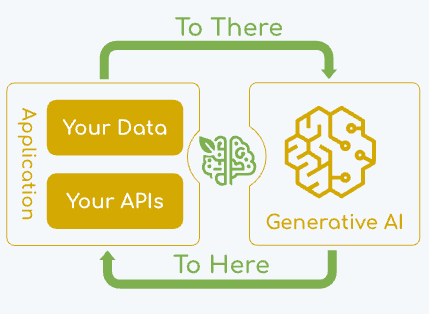
SpringAI聊天开发相关组件介绍和使用 Introduction and Usage of Spring AI Chat Components
介绍SpringAI的聊天组件ChatModel,相关概念和使用方法 An introduction to Spring AI's chat component ChatModel, related concepts and usage methods, along with code examples demonstrating its comprehensive usage.